SPF macros are placeholders used within SPF records. They dynamically dilate to specific values based on the characteristics of the email being processed, letting SPF mechanisms be more flexible and adaptive. If you have large-scale email systems, then we suggest you automate the management of SPF macros for efficient email authentication. For efficient management, consider using an automatic SPF flattening tool to handle SPF record resolution and avoid hitting DNS query limits. If you are interested in it, then read below to know how it can be done using scripting languages and APIs.
Understanding SPF macros and their relevance
SPF macros are character sequences used to replace metadata from emails that undergo SPF validation. Their proper use helps close security gaps, detect phishing attempts, and provide real-time information about who all sent emails on your behalf. They create precise and dynamic rules that contribute to validating email senders.
Here are the commonly integrated SPF macros–
- %{i}: Represents the IP address of the sender
- %{s}: Represents the sender’s email address (the “MAIL FROM” address).
- %{h}: Represents the HELO/EHLO domain of the SMTP server.
- %{d}: Represents the domain of the sender’s email address.
- %{p}: Represents the validated domain name of the sender’s IP address.
- %{v}: Represents the literal string “in-addr” or “ip6”, depending on whether the sender’s IP address is IPv4 or IPv6.
- %{l}: Represents the local part of the sender’s email address (the part before the “@” symbol).
- %{o}: Represents the domain part of the “MAIL FROM” address (the part after the “@” symbol).
- %{r}: Represents the domain of the recipient.
- %{t}: Represents the current timestamp in Unix time.
Define your requirements
Begin by making up your mind and identifying which domains and subdomains need an SPF record with macros. Then, document the IP ranges and third-party services that are officially allowed to send emails using these domains and subdomains.
What kind of macros are required depends on how complex your SPF records and email infrastructures are. Select a scripting language that you or the person you hired for the job is comfortable with. The commonly used ones are Python, Bash, and PowerShell. While Python is popular for its ease of use and vast library support, Bash is more suitable for UNIX-based systems with direct management via command-line tools like ‘dig’ and ‘nsupdate.’
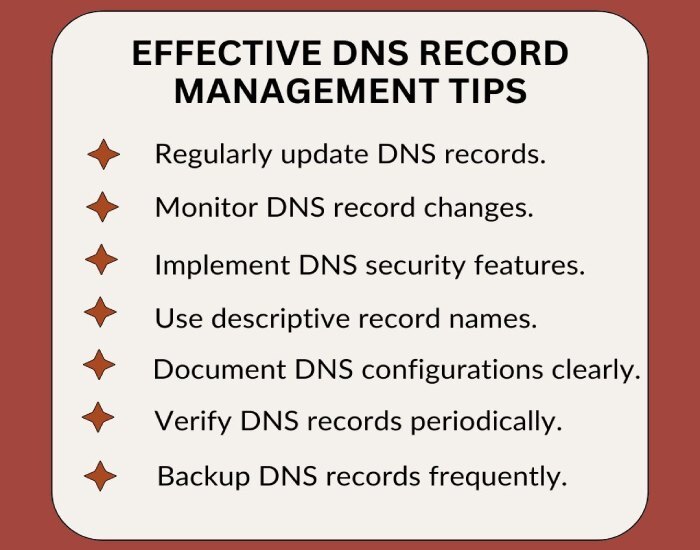
PowerShell, on the other hand, is recommended for Windows environments, as it comes with great scripting capabilities in DNS management.
Set up DNS API integration
Check with your DNS provider whether or not they have an option to offer an API for DNS record management. Once confirmed, proceed with API authentication within your scripts by obtaining keys or tokens. Don’t ignore knowing about the API endpoints for creating, updating, and deleting SPF records.
Script creation for SPF record management
Let’s understand this better by breaking it down into four steps.
- Fetching current SPF records: Write a script to fetch the current SPF records for your domains using the DNS API.
- SPF record parsing: Implement parsing logic to analyze existing SPF records, identifying macros and authorized IPs.
- Dynamic SPF record generation: Use the scripting language to dynamically generate or update SPF records based on current data (e.g., IP addresses, third-party services).
- API integration: Automate the update process by pushing the new SPF records to your DNS provider using their API.
The Python example shared below will be based on the following DNS API parameters–
api_url = "https://api.dnsprovider.com/v1/domains"
api_key = "your_api_key"
domain = "example.com"
The example starts here onwards-
# Fetch current SPF record
response = requests.get(f"{api_url}/{domain}/records", headers={"Authorization": f"Bearer {api_key}"})
spf_record = [record for record in response.json() if record['type'] == 'TXT' and 'v=spf1' in record['content']]
# Update SPF record dynamically
new_spf_record = "v=spf1 ip4:192.0.2.0/24 include:thirdparty.com ~all"
spf_record_id = spf_record[0]['id']
# Push updated SPF record to DNS
update_response = requests.put(
f"{api_url}/{domain}/records/{spf_record_id}",
headers={"Authorization": f"Bearer {api_key}"},
json={"content": new_spf_record}
print("SPF Record Updated:", update_response.status_code == 200)
Testing and validation
Once done, use an online SPF record lookup tool like Kitterman to know if everything is fine with your SPF record and that there are no syntactical and configurational errors. Verify that changes are correctly propagated across DNS servers and also send test emails to verify the SPF checks pass for different recipients.
Scheduling and monitoring
Set up cron jobs if you use Linux and a task scheduler if you use Windows to run your scripts at regular intervals. We recommend that you also implement logging and monitoring to track the success or failure of automated SPF updates. It’s also a good practice to set up alerts for issues.
Wrapping the process with error handling, documentation, and version control
Ensure your scripts handle API rate limits, authentication failures, and DNS update errors gracefully. Don’t forget to include failover mechanisms for situations where the primary DNS provider is not reachable.
Please maintain clear documentation of your scripts and include configuration details and dependencies. As for version control, use the right tool that allows you to track changes to your scripts and SPF records over time.